TransformationLayout
Transform into a different view or activity using morphing animations.
Using Transformation motions of new material version.
Including in your project
Gradle
Add below codes to your root build.gradle
file (not your module build.gradle file).
And add a dependency code to your module's build.gradle
file.
Usage
Add following XML namespace inside your XML layout file.
TransformationLayout
Here is a basic example of implementing TransformationLayout
.
We must wrap one or more views that we want to transform.
Transform into a view
Here is a simple example of transform fab into a view.
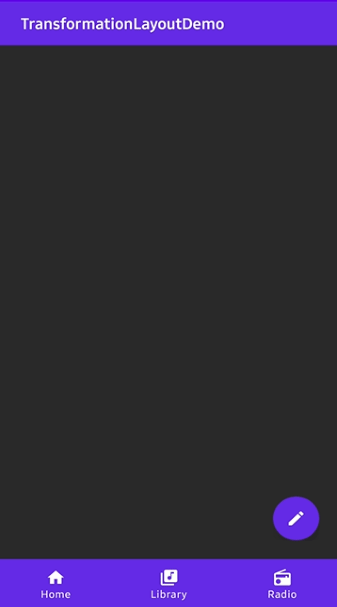
Bind a TargetView
We can bind a targetView that will be transformed from the TransformationLayout
using the below attribute in XML.
If you bind a targetView to the TransformationLayout
, the targetView's visibility will be GONE
.
Or we can bind a targetView using method.
Starting and finishing the transformation
After binding a targetView, we can start or finish transformation using the below methods.
The startTransform
and finishTransform
methods need a parent
as a parameter.
The parent parameter should be the root layout (the highest level layout).
Transform into an Activity

Here is an example of a transforming floating action button to Activity.
We don't need to bind a targetView.
onTransformationStartContainer
We should add onTransformationStartContainer()
to the Activity that has the floating action button. If your view is in the fragment, the code should be added to the fragment's Activity. It must be called before super.onCreate
.
Here is the Java way.
startActivity
And we should call startActivity
with bundle and intent data.
We should get a bundle
using withActivity
method. It needs a context and any name of transition.
The bundle
must be used as startActivity
's parameter.
We should put parcelable data to the intent using getParcelableParams()
method.
The extra name of the parcelable data can be anything, and it will be reused later.
If we want to get bundle data in RecyclerView or other classes,
we can use withView
and withContext
instead of withActivty
.
Here is the Java way.
onTransformationEndContainer
And finally, we should add onTransformationEndContainer()
to the Activity that will be started.
It must be added before super.onCreate
.
Here is the Java way.
OnTransformFinishListener
We can listen a TransformationLayout
is transformed or not using OnTransformFinishListener
.
Here is the Java way.
TransformationLayout Attributes
Attributes | Type | Default | Description |
---|---|---|---|
targetView | resource id | none | Bind a targetView that will be transformed. |
duration | Long | 350L | Duration of the transformation. |
pathMotion | Motion.ARC, Motion.LINEAR | default layout | Indicates that this transition should be drawn as the which path. |
containerColor | Color | Color.TRANSPARENT | Set the container color to be used as the background of the morphing container. |
scrimColor | Color | Color.TRANSPARENT | Set the color to be drawn under the morphing container. |
direction | Direction.AUTO, Direction.ENTER, Direction.RETURN | Direction.AUTO | Set the direction to be used by this transform. |
fadeMode | FadeMode.IN, FadeMode.OUT, FadeMode.CROSS, FadeMode.THROUGH | FadeMode.IN | Set the FadeMode to be used to swap the content of the start View with that of the end View. |
fitMode | FitMode.AUTO, FitMode.WIDTH, FitMode.HEIGHT | FitMode.AUTO | Set the fitMode to be used when scaling the incoming content of the end View. |
Find this library useful? :heart:
Support it by joining stargazers for this repository.