Carousel Recyclerview
Create carousel effect in recyclerview with the CarouselRecyclerview in a simple way.
Including in your project
Gradle
Add below codes to your root build.gradle
file (not your module build.gradle file).
allprojects {
repositories {
mavenCentral()
}
}
And add a dependency code to your module's build.gradle
file.
dependencies {
implementation 'com.github.sparrow007:carouselrecyclerview:1.2.1'
}
Usage
Basic Example for Kotlin
Here is a basic example of implementing carousel recyclerview in koltin files (activity or fragment) with attribute.
val carouselRecyclerview = findViewById<CarouselRecyclerview>(R.id.recycler)
carouselRecyclerview.adapter = adapter
Basic Example for XML
Here is a basic example of implementing carousel recyclerview in layout xml.
<com.jackandphantom.carouselrecyclerview.CarouselRecyclerview
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/recycler"/>
Property infinite and 3D on Item enabled | Property infinite and alpha on Item enabled |
---|---|
![]() |
![]() |
API Methods
Method | Description | Default |
---|---|---|
fun set3DItem(is3DItem: Boolean) | Make the view tilt according to their position, middle position does not tilt. | false |
fun setInfinite(isInfinite: Boolean) | Create the loop of the given view means there is no start or end, but provided position in the interface will be correct. | false |
fun setFlat(isFlat: Boolean) | Make the flat layout in the layout manager of the reyclerview | false |
fun setAlpha(isAlpha: Boolean) | Set the alpha for each item depends on the position in the layout manager | false |
fun setIntervalRatio(ratio: Float) | Set the interval ratio which is gap between items (views) in layout manager | 0.5f (value of gap, it should in range (0.4f - 1f)) |
fun getCarouselLayoutManager(): CarouselLayoutManager | Get the carousel layout manager instance | |
fun getSelectedPosition(): Int | Get selected position from the layout manager | center view Positoin |
API Methods Usage
val carouselRecyclerview = findViewById<CarouselRecyclerview>(R.id.recycler)
carouselRecyclerview.adapter = adapter
carouselRecyclerview.set3DItem(true)
carouselRecyclerview.setInfinite(true)
carouselRecyclerview.setAlpha(true)
carouselRecyclerview.setFlat(true)
val carouselLayoutManager = carouselRecyclerview.getCarouselLayoutManager()
val currentlyCenterPosition = carouselRecyclerview.getSelectedPosition()
Item Position Listener
You can listen to the position whenever the scroll happens you will get notified about the position, following are codes for listener
carouselRecyclerview.setItemSelectListener(object : OnSelected {
override fun onItemSelected(position: Int) {
//Cente item
}
})
Reflection ImageView

Use ReflectionImageView in xml layout and provide src
<com.jackandphantom.carouselrecyclerview.view.ReflectionImageView
android:layout_width="120dp"
android:layout_height="120dp"
android:scaleType="fitXY"
android:src="@drawable/hacker"
/>
Reflection Layout
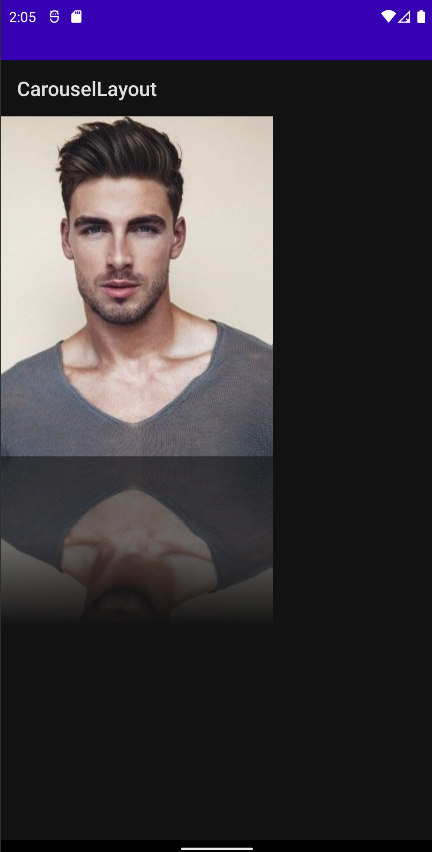
Now you can show reflection in more efficient way and 3x faster than ReflectionImageView see the codes below
<?xml version="1.0" encoding="utf-8"?>
<com.jackandphantom.carouselrecyclerview.view.ReflectionViewContainer
android:layout_width="wrap_content"
android:layout_height="wrap_content"
xmlns:android="http://schemas.android.com/apk/res/android"
app:reflect_relativeDepth="0.5"
app:reflect_gap="0dp"
xmlns:app="http://schemas.android.com/apk/res-auto"
>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/guypro"
android:scaleType="fitXY"
android:id="@+id/image" />
</com.jackandphantom.carouselrecyclerview.view.ReflectionViewContainer>
Notes about Reflection imageview
I would recommend you to use image loading library like Glide for loading image in reflection image for better performance
What's New Version 1.2.1 ??
Bugs Fix (ScrollToPosition)
Version 1.2.0
Adding reflection container
Version 1.1.0
Adding Support for orientation changes
Contribute ?
If you like the project and somehow wants to contribute, you are welcome to contribute by either submitting issues, refactor, pull request Thankyou.
Find this repository useful? ❤️
Support it by joining stargazers for this repository. :star:
And follow me for next creation ?
License
Copyright 2021 Sparrow007 (Ankit kumar)
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
GitHub
https://github.com/sparrow007/CarouselRecyclerview