A lightweight, simplified form validation library for Android
Screenshots
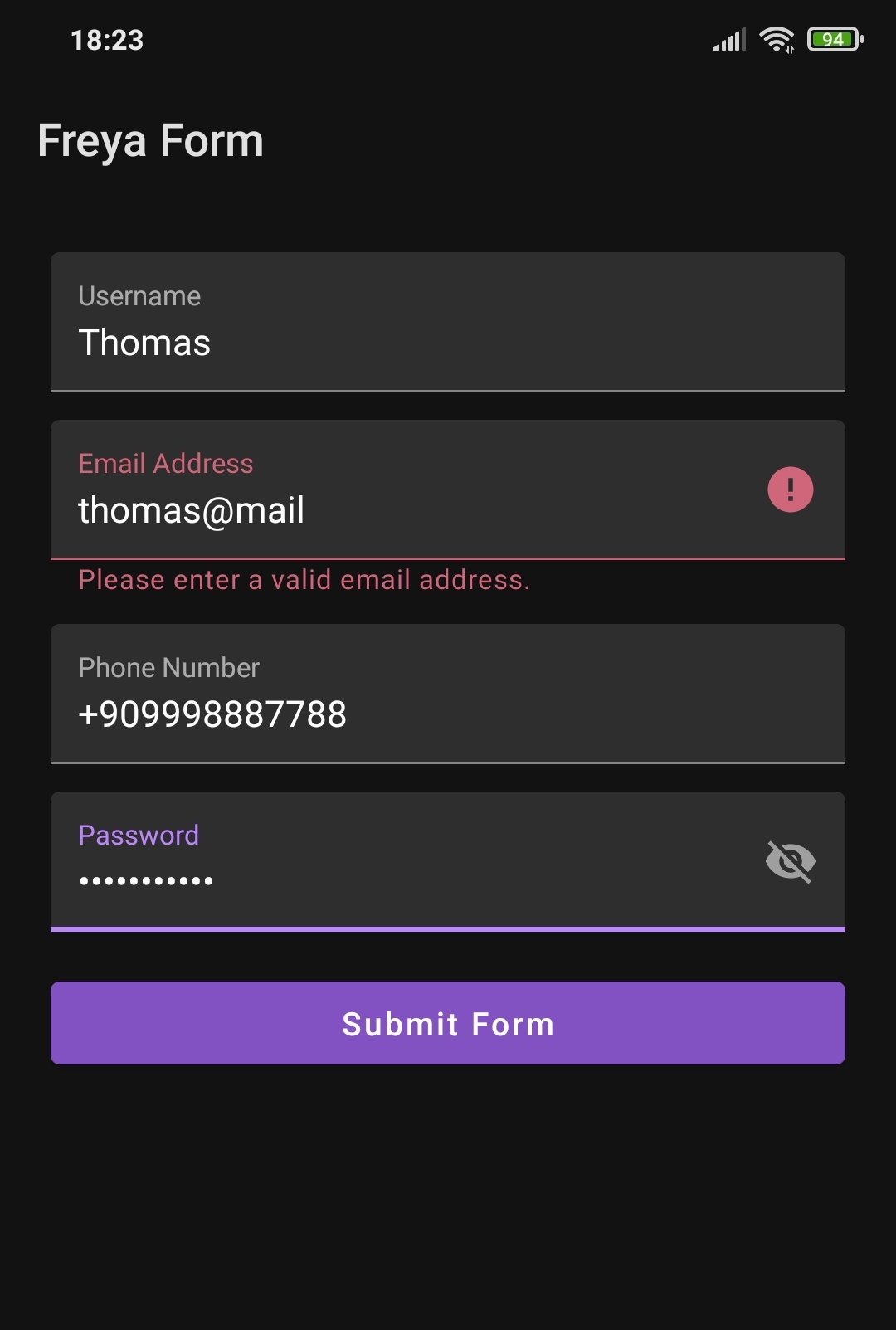
Validation Rules Setup
Form Layout Setup
Validation on Form Submit
Realtime Field Validation
Get Values & Prefill Form
Note
Currently supported field view types are TextInputLayout
, TextInputEditText
, EditText
.
Support for more view types and new validation rules will be added in the future.
Setup
Follow me on Twitter @ibrahimsn98
Step 1. Add the JitPack repository to your build file
Step 2. Add the dependency
GitHub
https://github.com/ibrahimsn98/Freya