Balloon
A lightweight popup like tooltips, fully customizable with arrow and animations.
Gradle
Add below codes to your root build.gradle
file (not your module build.gradle file).
And add a dependency code to your module's build.gradle
file.
Usage
Basic Example
Here is a basic example of implementing balloon popup with icon and text using Balloon.Builder
class.
Create using kotlin dsl
This is how to create Balloon
instance using kotlin dsl.
Show and dismiss
This is how to show balloon popup and dismiss.
Or you can show balloon popup using kotlin extension.
Arrow Composition
We can customize the arrow on the balloon popup.
Below previews are implemented using setArrowOrientation
and setArrowPosition
methods.
setArrowPosition
measures the balloon popup size and sets the arrow's position using the ratio value.
Orientation: BOTTOM Position: 0.62 showAlignTop |
Orientation: TOP Position : 0.5 showAlignBottom |
Orientation: LEFT Position: 0.5 showAlignRight |
Orientation: RIGHT Position: 0.5 showAlignLeft |
---|---|---|---|
![]() |
![]() |
![]() |
![]() |
Text Composition
We can customize the text on the balloon popup.
TextForm
TextFrom is an attribute class that has some attributes about TextView for customizing popup text.
This is how to create TextForm
using kotlin dsl.
Icon Composition
We can customize the icon on the balloon popup.
IconForm
IconForm is an attribute class that has some attributes about ImageView for customizing popup icon.
This is how to create IconForm
using kotlin dsl.
OnBalloonClickListener, OnBalloonDismissListener
We can listen to the balloon popup is clicked or dismissed using listeners.
We can simplify it using kotlin.
Customized layout
We can fully customize the ballloon layout using below method.
This is an example of implementing custom balloon popup.
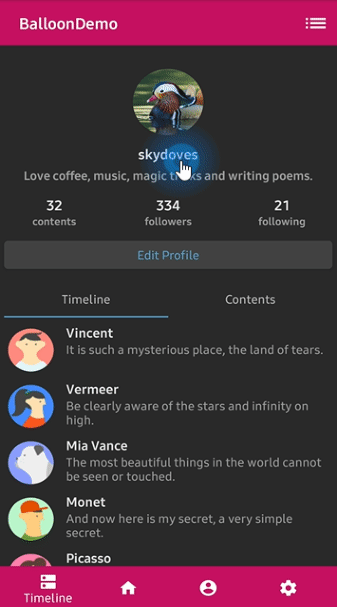
Firstly create an xml layout file like layout_custom_profile
on your taste.
And next we can get the inflated custom layout using getContentView
method.
Preference
If you want to show-up the balloon popup only once or a specific number of times, here is how to implement it simply.
But you can implement it more variously using Only.
Avoid Memory leak
Dialog, PopupWindow and etc.. have memory leak issue if not dismissed before activity or fragment are destroyed.
But Lifecycles are now integrated with the Support Library since Architecture Components 1.0 Stable released.
So we can solve the memory leak issue so easily.
Just use setLifecycleOwner
method. Then dismiss
method will be called automatically before activity or fragment would be destroyed.