Easings for Android
If you are not pleased with the native interpolators on Android. Here are 30 custom ones to make motion look more real.
This small library is a port of Robert Penner's easing equations to kotlin on Android to add flavors to your animations, and it's based on https://easings.net/
.
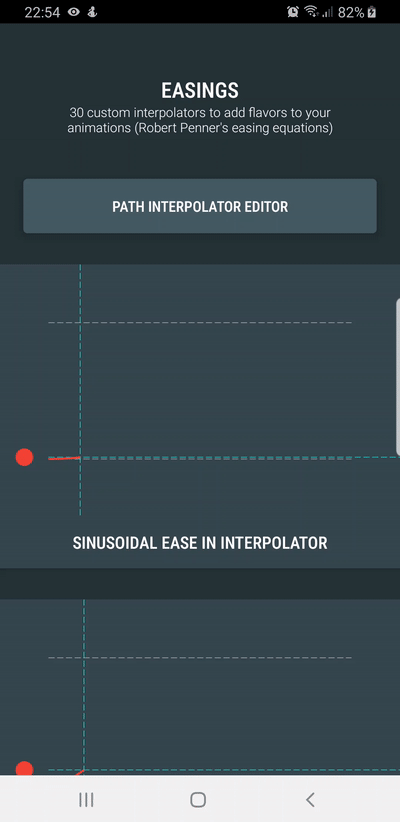
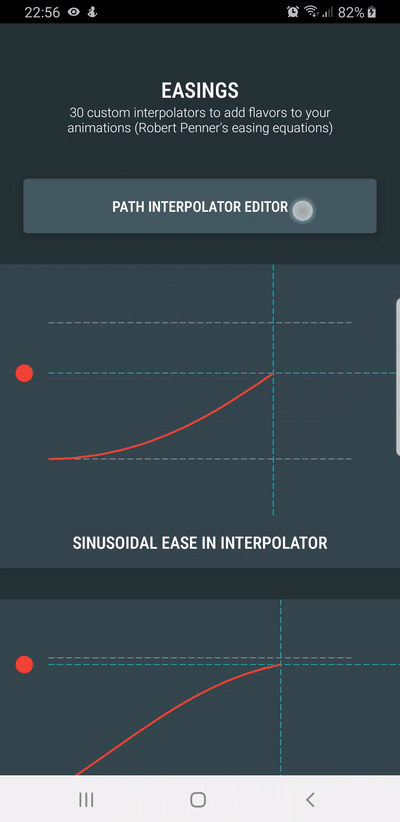
Until a demo gets released on Play, you can execute the sample and see the library's custom interpolators in action.
You can also experiment with the path interpolator editor to get the control points for a custom interpolator using PathInterpolatorCompat.create(x1, y1, x2, y2)
.
SETUP
Dependency should be declared in your app module level build.gradle
file:
dependencies {
implementation 'com.ramijemli:easings:1.0.0'
}
HOW TO USE
This can be used exactly like the native interpolators.
ValueAnimator.ofFloat(0f,1f).apply {
interpolator = Interpolators(Easings.SIN_IN)
start()
}
ObjectAnimator.ofFloat(textView, "translationX", 100f).apply {
interpolator = Interpolators(Easings.ELASTIC_IN_OUT)
start()
}
myView.animate().apply {
translationYBy(100f)
interpolator = Interpolators(Easings.BOUNCE_OUT)
start()
}
Interpolator | Behavior |
---|---|
Sinusoidal ease inEasings.SIN_IN |
![]() |
Sinusoidal ease outEasings.SIN_OUT |
![]() |
Sinusoidal ease in outEasings.SIN_IN_OUT |
![]() |
Quadratic ease inEasings.QUAD_IN |
![]() |
Quadratic ease outEasings.QUAD_OUT |
![]() |
Quadratic ease in outEasings.QUAD_IN_OUT |
![]() |
Cubic ease inEasings.CUBIC_IN |
![]() |
Cubic ease outEasings.CUBIC_OUT |
![]() |
Cubic ease in outEasings.CUBIC_IN_OUT |
![]() |
Quartic ease inEasings.QUART_IN |
![]() |
Quartic ease outEasings.QUART_OUT |
![]() |
Quartic ease in outEasings.QUART_IN_OUT |
![]() |
Quintic ease inEasings.QUINT_IN |
![]() |
Quintic ease outEasings.QUINT_OUT |
![]() |
Quintic ease in outEasings.QUINT_IN_OUT |
![]() |
Exponential ease inEasings.EXP_IN |
![]() |
Exponential ease outEasings.EXP_OUT |
![]() |
Exponential ease in outEasings.EXP_IN_OUT |
![]() |
Circular ease inEasings.CIRC_IN |
![]() |
Circular ease outEasings.CIRC_OUT |
![]() |
Circular ease in outEasings.CIRC_IN_OUT |
![]() |
Back ease inEasings.BACK_IN |
![]() |
Back ease outEasings.BACK_OUT |
![]() |
Back ease in outEasings.BACK_IN_OUT |
![]() |
Elastic ease inEasings.ELASTIC_IN |
![]() |
Elastic ease outEasings.ELASTIC_OUT |
![]() |
Elastic ease in outEasings.ELASTIC_IN_OUT |
![]() |
Bounce ease inEasings.BOUNCE_IN |
![]() |
Bounce ease outEasings.BOUNCE_OUT |
![]() |
Bounce ease in outEasings.BOUNCE_IN_OUT |
![]() |
CONTRIBUTION
All bugs, feature requests, feedback, etc. are welcome. Please, feel free to create an issue.
If you have new ideas, feel free to contribute by opening pull requests on dev branch.
APPS USING IT
Are you using this library in your app? Let us know and we'll show it here.
TO DO
- [ ] Add tension modifier for elastic easing
- [ ] Add path interpolator editor
- [ ] Add sample for google play