The simplest navigation library for Android
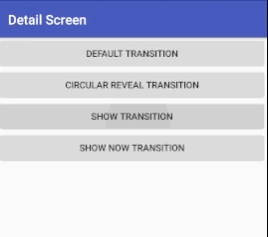
Magellan
The simplest navigation library for Android.
Main Features
- Navigation is as simple as calling
goTo(screen)
- You get full control of the backstack
- Transitions are automaticaly handled for you
Download
Add the dependencies you need in your build.gradle
:
Core library
compile 'com.wealthfront:magellan:1.0.0'
Optional add-ons
def magellanVersion = '1.0.0'
compile 'com.wealthfront:magellan:' + magellanVersion
compile 'com.wealthfront:magellan-support:' + magellanVersion
compile 'com.wealthfront:magellan-rx:' + magellanVersion
Add-on coming soon
- Rx 2: already merged, will be in the next release (thanks to @FabianTerhorst).
- Design lib (for tabs), in the meantime, here is the code to implement tabs.
Getting started
Single Activity
MainActivity.java
:
public class MainActivity extends SingleActivity {
@Override
protected Navigator createNavigator() {
return Navigator.withRoot(new HomeScreen()).build();
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
activity_main.xml
:
<com.wealthfront.magellan.ScreenContainer
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/magellan_container"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
Minimal Screen implementation
Screen example HomeScreen.java
:
public class HomeScreen extends Screen<HomeView> {
@Override
protected HomeView createView(Context context) {
return new HomeView(context);
}
}
Associated View HomeView.java
:
public class HomeView extends BaseScreenView<HomeScreen> {
public HomeView(Context context) {
super(context);
inflate(context, R.layout.home, this);
}
}