The button which can use with icon and text
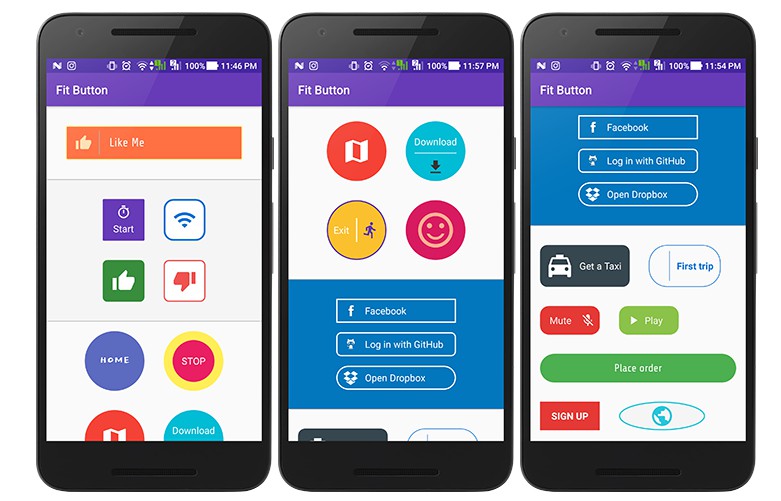
# FitButton
The button which can use with icon, text, divider, custom ripple effect, border, corner radius e.t.c.
The button which includes features:
- Icon,
- Text,
- Divider,
- Corner radius,
- Border of the button,
- Custom ripple effect,
- Custom elements sizes,
- Custom colors of elements,
- Different positions of elements on the button
Installation
Gradle
implementation 'com.github.nikartm:fit-button:1.1.1'
Screenshots
How to use?
Adjust the XML view:
<com.github.nikartm.button.FitButton
android:id="@+id/fbtn"
android:layout_width="match_parent"
android:layout_height="58dp"
android:layout_margin="32dp"
android:enabled="true"
app:fb_cornerRadius="2dp"
app:fb_shape="rectangle"
app:fb_divColor="#fff"
app:fb_divWidth="1dp"
app:fb_divHeight="40dp"
app:fb_rippleColor="#FBE9E7"
app:fb_iconWidth="28dp"
app:fb_iconHeight="28dp"
app:fb_iconPosition="left"
app:fb_iconMarginStart="30dp"
app:fb_iconMarginEnd="16dp"
app:fb_icon="@drawable/ic_thumb_up"
app:fb_iconColor="#FBE9E7"
app:fb_textPaddingStart="16dp"
app:fb_textColor="#FFF"
app:fb_textGravity="start|center"
app:fb_text="Like Me"
app:fb_backgroundColor="#FF7043"/>
Or programmatically:
private fun setupButton() {
fitButton = findViewById(R.id.fbtn)
fitButton!!
.setTextFont(R.font.share_tech_regular)
.setTextSize(20f)
.setIconMarginStart(16f)
.setIconMarginEnd(12f)
.setTextColor(Color.parseColor("#F5F5F5"))
.setIconColor(Color.parseColor("#FFE0B2"))
.setDividerColor(Color.parseColor("#BCAAA4"))
.setBorderColor(Color.parseColor("#FFF59D"))
.setButtonColor(Color.parseColor("#FF7043"))
.setBorderWidth(2f)
.setRippleEnable(true)
.setRippleColor(resources.getColor(R.color.colorAccent))
.setOnClickListener {
Toast.makeText(this,
"Click on ${fitButton?.getText()}",
Toast.LENGTH_SHORT).show()
}
}
Kotlin support
If you got an error
Caused by: java.lang.NoClassDefFoundError: Failed resolution of: Lkotlin/jvm/internal/Intrinsics;
You may need to add Kotlin support to your project.
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
and add to the root build.gradle
buildscript {
ext.kotlin_version = 'X.Y.Z'
...
}