Expandable Bottom Bar improve navigation in your app
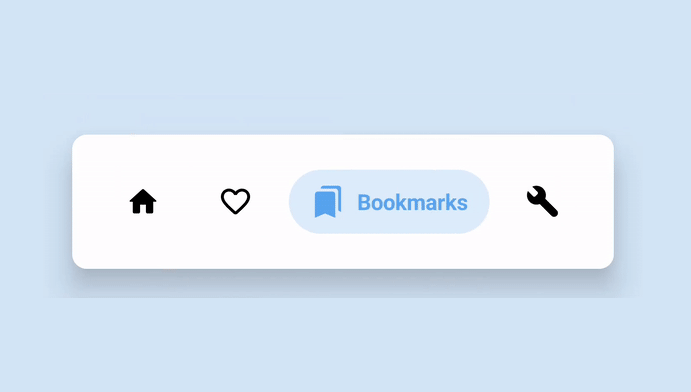
ExpandableBottomBar
A new way to improve navigation in your app
Its really easy integrate to your project
take it, faster, faster
- Maven
<dependency>
<groupId>com.github.st235</groupId>
<artifactId>expandablebottombar</artifactId>
<version>X.X</version>
<type>pom</type>
</dependency>
- Gradle
implementation 'com.github.st235:expandablebottombar:X.X'
- Ivy
<dependency org='com.github.st235' name='expandablebottombar' rev='X.X'>
<artifact name='expandablebottombar' ext='pom' ></artifact>
</dependency>
P.S.: Check out latest version code in badge at the top of this page.
Usage
Really simple as I wrote earlier
Firstly, you should declare your view in xml file
<github.com.st235.lib_expandablebottombar.ExpandableBottomBar
android:id="@+id/expandable_bottom_bar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="20dp"
app:exb_backgroundCornerRadius="25dp"
app:exb_backgroundColor="#2e2e2e"
app:exb_itemInactiveColor="#fff"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
Then you should add menu items to your navigation component
val bottomBar: ExpandableBottomBar = findViewById(R.id.expandable_bottom_bar)
bottomBar.addItems(
ExpandableBottomBarMenuItem.Builder(context = this)
.addItem(R.id.icon_home, R.drawable.ic_bug, R.string.text, Color.GRAY)
.addItem(R.id.icon_go, R.drawable.ic_gift, R.string.text2, 0xFFFF77A9)
.addItem(R.id.icon_left, R.drawable.ic_one, R.string.text3, 0xFF58A5F0)
.addItem(R.id.icon_right, R.drawable.ic_two, R.string.text4, 0xFFBE9C91)
.build()
)
bottomBar.onItemSelectedListener = { view, menuItem ->
/**
* handle menu item clicks here,
* but clicks on already selected item will not affect this callback
*/
}
bottomBar.onItemReselectedListener = { view, menuItem ->
/**
* handle here all the click in already selected items
*/
}
Xml menu declaration
If your menu is constantly, you may specify it from xml
Firstly, you should declare menu items in xml
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/home"
android:title="@string/text"
app:exb_color="#FF8888"
app:exb_icon="@drawable/ic_home" />
<item
android:id="@+id/settings"
android:title="@string/text4"
app:exb_color="@color/colorSettings"
app:exb_icon="@drawable/ic_settings" />
<item
android:id="@+id/bookmarks"
android:title="@string/text3"
app:exb_color="#fa2"
app:exb_icon="@drawable/ic_bookmarks" />
</menu>
each item tag has following attributes:
property | type | description |
---|---|---|
id | reference | an id of menu item |
color | reference/color | color of element, it may be color reference or color |
icon | reference | icon reference (vector drawables supported) |
title | reference/text | item title |
Just like any Android menu ?
Then you should reference this xml file at the view attributes
<github.com.st235.lib_expandablebottombar.ExpandableBottomBar
android:id="@+id/expandable_bottom_bar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="20dp"
app:exb_backgroundCornerRadius="25dp"
app:exb_itemInactiveColor="#fff"
app:exb_items="@menu/bottom_bar"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
Xml attributes
property | type | description |
---|---|---|
exb_elevation | dimen | component elevation (important: api 21+) |
exb_backgroundColor | color | bottom bar background color |
exb_transitionDuration | integer | time between one item collapsed and another item expanded |
exb_backgroundCornerRadius | dimen | bottom bar background corners radius |
exb_itemInactiveColor | color | item menu color, when its inactive |
exb_itemBackgroundCornerRadius | dimen | item background corner radius |
exb_itemBackgroundOpacity | float | item background opacity (important: final color alpha calculates by next formulae alpha = opacity * 255) |
exb_item_vertical_margin | dimen | top & bottom item margins |
exb_item_horizontal_margin | dimen | left & right item margins |
exb_item_vertical_padding | dimen | top & bottom item padding |
exb_item_horizontal_padding | dimen | left & right item padding |
exb_items | reference | xml supported menu format |
Coordinator Layout support
Do you waiting for Coordinator Layout support - and it is already here! Fabs and Snackbars aligned by bottom bar! Hooray ?
Available without registration and SMS, starting from 0.8 version. Seriously, everything is already working out of the box - nothing needs to be done.
But... if you need to support hiding the menu by list/grid scroll - then you are really lucky!
This functionality is very simple to implement. You need to redeclare custom Coordinator Layout Behavoir
to ExpandableBottomBarScrollableBehavior
.
<github.com.st235.lib_expandablebottombar.ExpandableBottomBar
android:id="@+id/expandable_bottom_bar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
app:layout_behavior="github.com.st235.lib_expandablebottombar.behavior.ExpandableBottomBarScrollableBehavior"
app:items="@menu/bottom_bar" />
Really easy ;D
After integration this behavior should looks like:
Migration guide
You may found all necessary info about migration from old versions here