Bottom Navigation based on Bottom Navigation View from Android
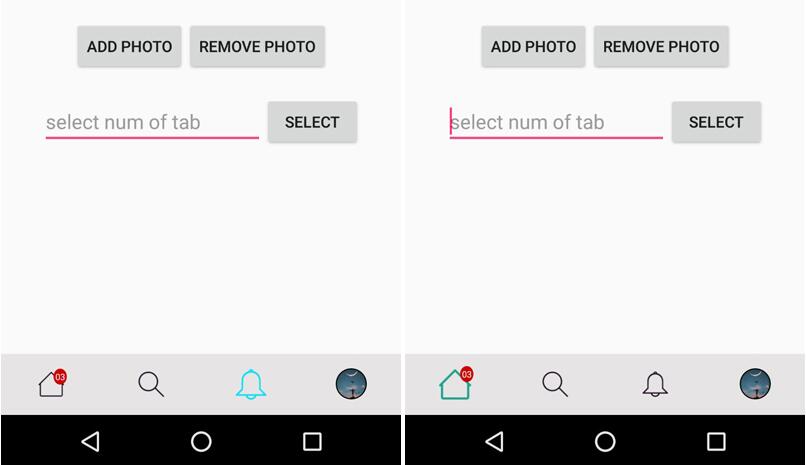
BottomNavygation
Bottom Navigation based on Bottom Navigation View from Android.
Getting Started
Add it in your root build.gradle (Project module)
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Add the dependency in build.gradle (App module)
dependencies {
compile 'com.github.felixsoares:BottomNavygation:1.8.6'
}
Usage example
In layout file
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.felix.bottomnavygation.BottomNav
android:id="@+id/bottomNav"
android:background="@color/gray"
android:layout_alignParentBottom="true"
android:layout_width="match_parent"
android:layout_height="55dp"/>
</RelativeLayout>
In Activity or Fragment
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.explore, "Explore").addColorAtive(R.color.colorAccent));
bottomNav.addItemNav(new ItemNav(this, R.mipmap.atividades).addColorAtive(R.color.colorAccent));
bottomNav.build();
Documentation
- Support click and longClick listeners (just in case if ItemNav is profile item).
bottomNav.setTabSelectedListener(listener);
BottomNav.OnTabSelectedListener listener = new BottomNav.OnTabSelectedListener() {
@Override
public void onTabSelected(int position) {
Toast.makeText(MainActivity.this, "Click position " + position, Toast.LENGTH_SHORT).show();
}
@Override
public void onTabLongSelected(int position) {
Toast.makeText(MainActivity.this, "Long position " + position, Toast.LENGTH_SHORT).show();
}
};
- Support to add badge in ItemNav.
BadgeIndicator badgeIndicator = new BadgeIndicator(this, android.R.color.holo_red_dark, android.R.color.white);
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.feed).addColorAtive(R.color.colorAccent).addBadgeIndicator(badgeIndicator));
bottomNav.build();
- Update badge.
badgeIndicator.updateCount(count);
- Support to add profile photo int ItemNav.
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.perfil).addColorAtive(R.color.colorAccent).setPathImageProfile(YOUR_IMAGE_PATH));
bottomNav.build();
- Support to ative and inative colors in tab.
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.explore).addColorAtive(R.color.colorAccent).addColorInative(R.color.colorPrimary));
bottomNav.build();
- Support to select especific Tab.
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.explore));
bottomNav.build();
bottomNav.selectTab(0);
- Support to change icon when is ative.
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(this, R.mipmap.explore, R.mipmap.explore_ative));
bottomNav.build();
- Support to change color of border from profile photo when is ative or inative.
BottomNav bottomNav = findViewById(R.id.bottomNav);
bottomNav.addItemNav(new ItemNav(contexto, R.drawable.explore, R.drawable.explore_sel).isProfileItem().addProfileColorAtive(R.color.verdepadrao).addProfileColorInative(R.color.preto));
bottomNav.build();