Android View for displaying piano chord and scale charts in music theory apps for pianists
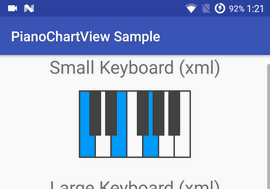
Piano Chart View
Elementary and clean Android View for displaying piano chord and scale charts in music theory apps for pianists.
Sample
Installation
Step 1
Add the JitPack repository to your build file
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Step 2
Add the dependency
dependencies {
compile 'com.github.Andy671:PianoChartView:v0.6.6'
}
Usage
Creating from xml:
In your layout.xml
xmlns:custom="http://schemas.android.com/apk/res-auto"
<!-- Custom arguments are optional - if you don't override them it uses default values -->
<com.kekstudio.pianochartview.PianoChartView
android:id="@+id/piano_chart_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
custom:checkedKeysColor="@android:color/white"
custom:lightKeysColor="@color/colorCustomLight"
custom:darkKeysColor="@color/colorCustomDark"
custom:size="Small"
custom:checkedKeys="@array/some_integer_array"/>
In your arrays.xml
<!-- Numbers = keys on keyboard -->
<integer-array name="some_integer_array">
<item>5</item>
<item>7</item>
<item>11</item>
<item>1</item>
</integer-array>
In your colors.xml
<color name="colorCustomLight">#C8E6C9</color>
<color name="colorCustomDark">#1B5E20</color>
Editing from code:
PianoChartView pianoChartView = (PianoChartView) findViewById(R.id.piano_chart_view_small);
pianoChartView.setCheckedKeys(new int[]{2, 5, 3, 8, 11, 12});
pianoChartView.setSize(PianoChartView.Size.Small);
pianoChartView.setLightKeysColor(Color.parseColor("#CFD8DC"));
pianoChartView.setDarkKeysColor(Color.parseColor("#607D8B"));
pianoChartView.setCheckedKeysColor(Color.parseColor("#B2EBF2"));
See sample for more info
XML Attributes
Attribute | Type | Default |
---|---|---|
lightKeysColor | color | Color.WHITE |
darkKeysColor | color | Color.DKGRAY |
checkedKeysColor | color | #03A9F4 |
additionalCheckedKeysColor | color | #03A9F4 |
checkedKeys | reference (int[]) | { } |
additionalCheckedKeys | reference (int[]) | { } |
namesOfKeys | reference (String[]) | { } |
size | enum [Large, Small] | Large |
Public methods
Type | Method |
---|---|
void | setCheckedKeys(int[] numbers) |
void | setAdditionalCheckedKeys(int[] numbers) |
void | setNamesOfKeys(String... keyLetters) |
void | setSize(Size size) |
void | setLightKeysColor(int color) |
void | setDarkKeysColor(int color) |
void | setCheckedKeysColor(int color) |
void | setAdditionalCheckedKeysColor(int color) |
int[] | getCheckedKeys() |
int[] | getAdditionalCheckedKeys() |
Size | getSize() |
int | getLightKeysColor() |
int | getDarkKeysColor() |
int | getCheckedKeysColor() |
int | getAdditionalCheckedKeysColor() |