A TextView that shows the right text based on its size
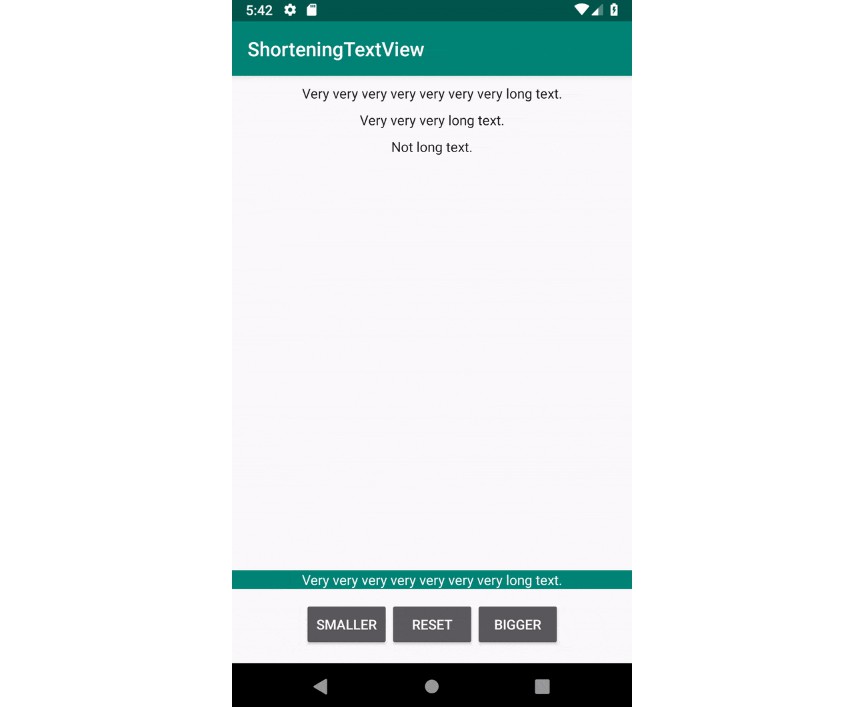
ShorteningTextView
A TextView that shows the right text based on its size!
Installation
Add the following line in the dependencies
section of your build.gradle
file:
implementation 'com.alexfacciorusso.shorteningtextview:shorteningtextview:0.1.0'
Usage
Use the com.alexfacciorusso.shorteningtextview.ShorteningTextView
view into your layouts.
<com.alexfacciorusso.shorteningtextview.ShorteningTextView
android:id="@+id/shorteningTextView"
app:stv_texts="@array/example_strings"
... />
where @array/exampe_strings
is an array of strings defined as a resource.
If you want to set the texts in a programmatic way (we all love setting views programmatically,
don't we?), a property texts
(Kotlin) or setTexts/getTexts
(Java) is available!
Kotlin
val sampleStrings = resources.getStringArray(R.array.example_strings)
// Notice the `.toList()` since `texts` is a List<String> but Resources.getStringArray
// returns, of course, an array.
shorteningTextView.texts = sampleStrings.toList()
// Or also:
shorteningTextView.texts = listOf("My very long string", "My string")
Java
final String[] sampleTexts = getResources().getStringArray(R.array.example_strings);
// Notice the `Arrays.asList` since `texts` is a List<String> but Resources.getStringArray
// returns, of course, an array.
shorteningTextView.setTexts(Arrays.asList(sampleTexts));