A simple library to add dynamic and pinned shortcuts
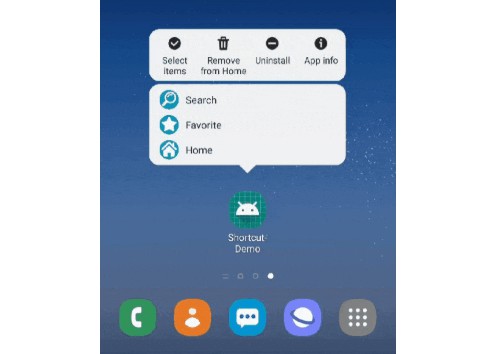
Shortcut
A simple library to add dynamic and pinned shortcuts.
Integrating the shortcut sdk into your android app
Add jitpack maven repo to app module's build.gradle
allprojects {
repositories {
maven { url "https://jitpack.io" }
}
}
maven
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
Add dependency
gradle
dependencies {
implementation 'com.github.MehdiKh93:Shortcut:1.0.2'
}
maven
<dependency>
<groupId>com.github.MehdiKh93</groupId>
<artifactId>Shortcut</artifactId>
<version>1.0.2</version>
</dependency>
Usage
init ShortcutUtils
class
ShortcutUtils shortcutUtils = new ShortcutUtils(context);
adding a DynamicShortcut
Shortcut dynamicShortcut = new Shortcut.ShortcutBuilder()
.setShortcutIcon(R.drawable.icon)
.setShortcutId("dynamicShortcutId")
.setShortcutLongLabel("dynamicShortcutLongLable")
.setShortcutShortLabel("dynamicShortcutShortLabel")
.setIntentAction("dynamicShortcutIntentAction")
.setIntentStringExtraKey("dynamicShortcutKey")
.setIntentStringExtraValue("dynamicShortcutValue")
.build();
shortcutUtils.addDynamicShortCut(dynamicHomeShortcut, new IReceiveStringExtra() {
@Override
public void onReceiveStringExtra(String stringExtraKey, String stringExtraValue) {
String intent = getIntent().getStringExtra(stringExtraKey);
if (intent != null) {
if (intent.equals("dynamicShortcutValue")) {
//write any code here
}
}
}
});
}
disabling a DynamicShortcut
temporary
shortcutUtils.disableDynamicShortCut(dynamicShortcut);
removing a DynamicShortcut
temporary
shortcutUtils.removeDynamicShortCut(dynamicShortcut);
enabling a DynamicShortcut
temporary
shortcutUtils.enableDynamicShortCut(dynamicShortcut);
initing a PinnedShortcut
Shortcut pinnedShortcut = new Shortcut.ShortcutBuilder()
.setShortcutIcon(R.drawable.icon)
.setShortcutId("pinnedShortcutId")
.setShortcutLongLabel("pinnedShortcutLongLabel")
.setShortcutShortLabel("pinnedShortcutShortLabel")
.setIntentAction("pinnedShortcutIntentAction")
.setIntentStringExtraKey("pinnedShortcutKey")
.setIntentStringExtraValue("pinnedShortcutValue")
.build();
shortcutUtils.initPinnedShortCut(pinnedShortcut, new IReceiveStringExtra() {
@Override
public void onReceiveStringExtra(String stringExtraKey, String stringExtraValue) {
String intent = getIntent().getStringExtra(stringExtraKey);
if (intent != null) {
if (intent.equals("pinnedShortcutValue")) {
//write any code here
}
}
}
});
}
requesting a PinnedShortcut
shortcutUtils.requestPinnedShortcut(pinnedShortcut);
disabling a PinnedShortcut
shortcutUtils.disablePinnedShortCut(pinnedShortcut);
enabling a PinnedShortcut
shortcutUtils.enablePinnedShortCut(pinnedShortcut);
Issues
Please send all issues and feedback to khalifeh.mehdi@gmail.com or Telegram ID: https://t.me/mehdikhalifeh