A RecyclerView with layout animations
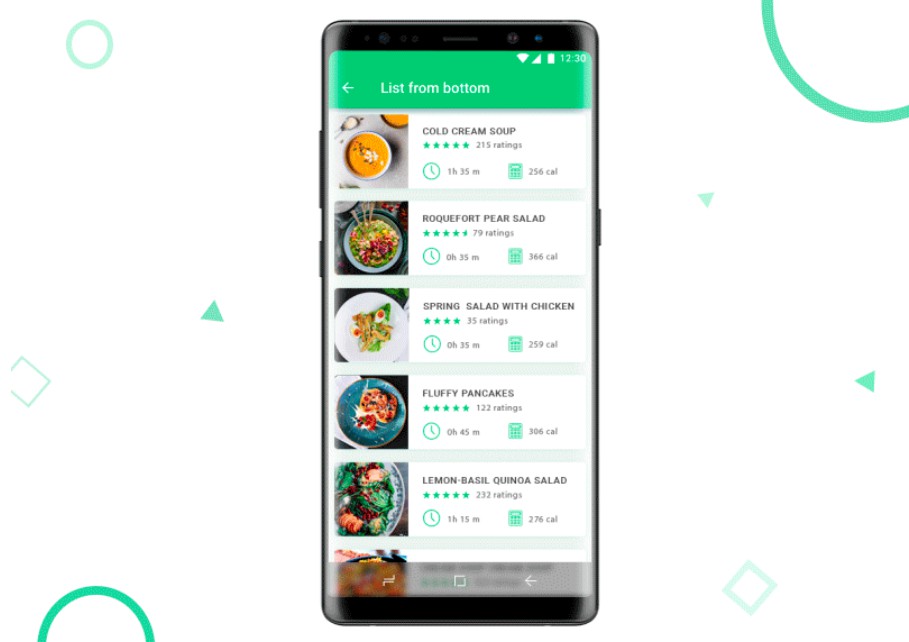
AnimatedRecyclerView
A RecyclerView with layout animations.
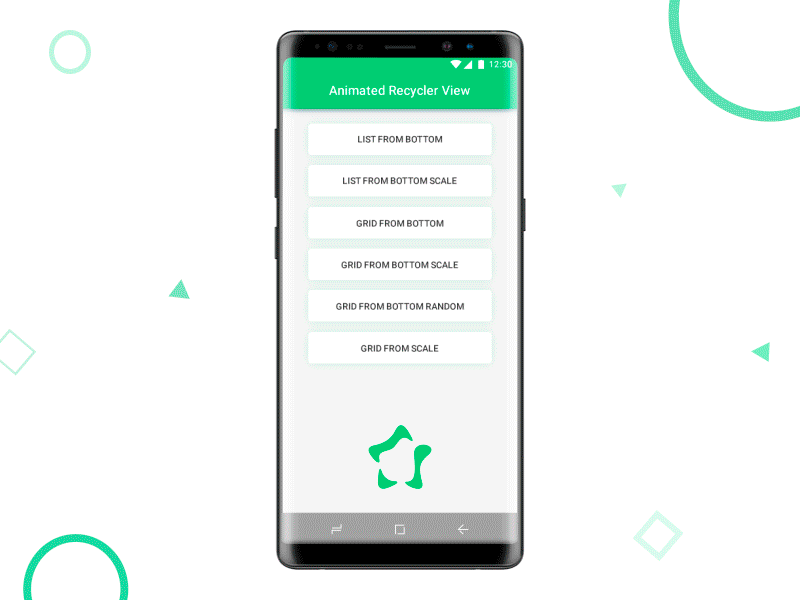
Setup
To use this library your minSdkVersion
must be >= 16.
In your build.gradle :
dependencies {
implementation "com.mlsdev.animatedrv:library:1.0.1"
}
Example
From the layout
<com.mlsdev.animatedrv.AnimatedRecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:animationDuration="600"
app:layoutAnimation="@anim/layout_animation_from_bottom"
app:layoutManagerOrientation="vertical"
app:layoutManagerReverse="false"
app:layoutManagerType="linear" />
You can create any layout animations
<layoutAnimation xmlns:android="http://schemas.android.com/apk/res/android"
android:animation="@anim/item_animation_from_bottom"
android:animationOrder="normal"
android:delay="15%" />
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:duration="500">
<translate
android:fromYDelta="50%p"
android:interpolator="@android:anim/accelerate_decelerate_interpolator"
android:toYDelta="0" />
<alpha
android:fromAlpha="0"
android:interpolator="@android:anim/accelerate_decelerate_interpolator"
android:toAlpha="1" />
</set>
From the code
AnimatedRecyclerView recyclerView = new AnimatedRecyclerView.Builder(this)
.orientation(LinearLayoutManager.VERTICAL)
.layoutManagerType(AnimatedRecyclerView.LayoutManagerType.LINEAR)
.animation(R.anim.layout_animation_from_bottom)
.animationDuration(600)
.reverse(false)
.build();
Start animation
- First option
adapter.notifyDataSetChanged();
recyclerView.scheduleLayoutAnimation();
- Second option
YourRecyclerView
must be casted to theAnimatedRecyclerView
and an adapter must be set.
recyclerView.notifyDataSetChanged();