A library for image manipulation with power of renderScript
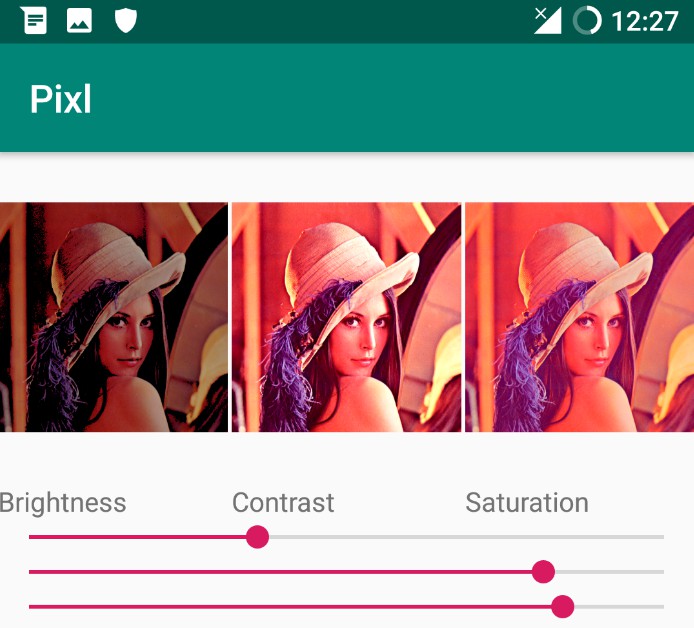
Pixl
Pixl is a library for image manipulation with power of renderScript which is faster than other ordinary solutions, currently it includes three basic scripts, brightness, contrast, saturation.
Installation
Add Jitpack to your project build.gradle file
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
jcenter()
}
}
}
Then add this dependency to your app build.gradle file.
dependencies {
implementation 'com.jibraniqbal.pixl:pixl:0.0.1'
}
Usage
You can see the example with brightness, contrast, saturation with seekbar, given the value 0-100%
Add support for API-19 add this to you build.gradle
android {
defaultConfig {
...
renderscriptTargetApi 19
renderscriptSupportModeEnabled true
}
}
Intialization
val pixl = Pixl(context)
Brightness
brightnessSeek.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener {
override fun onProgressChanged(p0: SeekBar?, p1: Int, p2: Boolean) {
val input = originalBrightnessImage
val output = Bitmap.createBitmap(input.width, input.height, input.config)
pixl.setBrightness(input, output, p0?.progress?.toFloat() ?: 0f)
postImage(brightnessImage, output)
}
override fun onStartTrackingTouch(p0: SeekBar?) {}
override fun onStopTrackingTouch(p0: SeekBar?) {}
})
Contrast
contrastSeek.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener {
override fun onProgressChanged(p0: SeekBar?, p1: Int, p2: Boolean) {
val input = originalContrastImage
val output = Bitmap.createBitmap(input.width, input.height, input.config)
pixl.setContrast(input, output, p0?.progress?.toFloat() ?: 0f)
postImage(contrastImage, output)
}
override fun onStartTrackingTouch(p0: SeekBar?) {}
override fun onStopTrackingTouch(p0: SeekBar?) {}
})
Saturation
saturationSeek.setOnSeekBarChangeListener(object : SeekBar.OnSeekBarChangeListener {
override fun onProgressChanged(p0: SeekBar?, p1: Int, p2: Boolean) {
val input = originalSaturationImage
val output = Bitmap.createBitmap(input.width, input.height, input.config)
pixl.setSaturation(input, output, p0?.progress?.toFloat() ?: 0f)
postImage(saturationImage, output)
}
override fun onStartTrackingTouch(p0: SeekBar?) {}
override fun onStopTrackingTouch(p0: SeekBar?) {}
})